Qt based editor for GLFW based game engine
- Shaveen Kumar
- May 2, 2017
- 2 min read
I spent quite a bit of time trying to figure out what would be the best way to have an Editor for my game engine (which I ended up renaming to Nexus). After doing some research and asking around, I ending up deciding to go with a Qt based editor. The biggest challenge was trying to get everything to function in a seamless manner with minimal additional code. The Nexus Engine makes use of GLFW to create the OpenGL context and I use GLM for linear algebra needed for the engine. Qt has its own classes for vectors, matrices, quaternions, etc. Aside from that, it also has quite a few classes to create an OpenGL context. After reading about it a little, I chose to go with QOpenGLWidget as this will help me make use of the QWidget capabilities I need in the editor and also, it has support for newer OpenGL API. The Qt Creator comes with some great examples that helped me get started. I tried getting some basic OpenGL code running in a simple editor context. After I was able to do this, I had confidence that with an unknown amount of effort, I should surely be able to build the kind of editor I have in mind. Figuring out how much effort it would take was tricky.
What I ended up doing initially was making use of most of the math classes from Qt (QVector, QMatrix4x4, etc.). By doing this, I ended up with a whole bunch of #ifdef blocks in my code that were trying to separate out the GLFW and GLM specific bits from the Qt ones. Ugh. The problem was that at this time, I wasn't sure if I was able to even get my render pipeline working with the Qt context without going through the process of completely "Qt-fying" my engine. I did manage to not have to retype too much of the code as I use typedefs for pretty much all non standard datatypes so I just had to define these for my Qt pipeline. After going down this road far enough, I started to realize how messy everything was starting to get so I had to look for another way around this. Also, several gl function calls (like glBufferData()) that I used in my engine were not supported by even the newer QtOpenGLFunctions class. It was at this point I decided that I have to try and override this functionality if I wanted to keep up with the newer versions of OpenGL. Thankfully this wasn't too hard to do. By using glew(OpenGL Extension Wrangler) libraries, I was able to easily override what was provided by QtOpenGLFunctions and by doing this, everything started to "just work". I was able to revert to using GLM instead of the Qt helper classes. At the end of the restructure, I was able to get rid of almost all the #ifdefs and I had my engine running perfectly within the confines of an editable QMainWindow to which I'm going to add all my menu and toolbar functionality. This is what I'm starting off with:
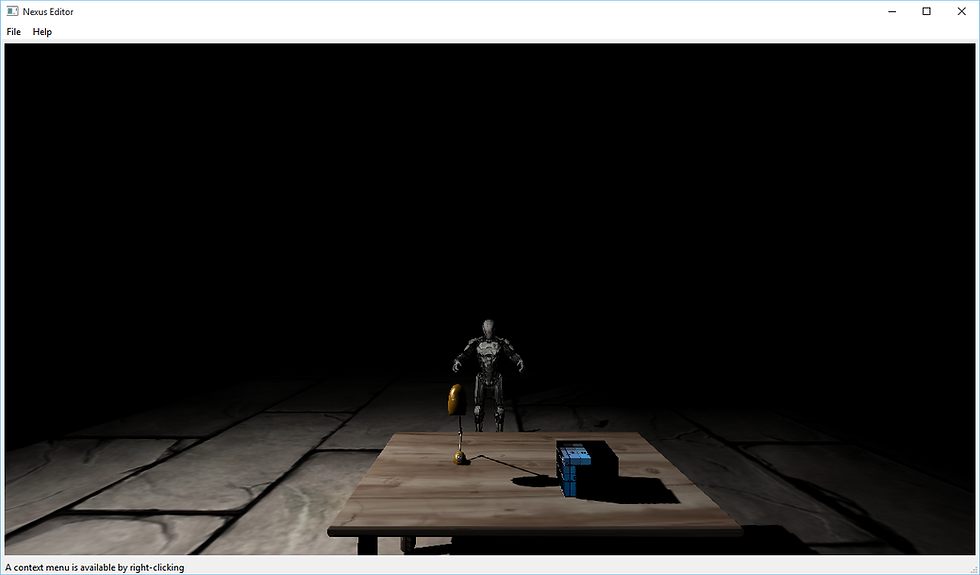
Comments